Unlock Innovation
Explore the latest tech trends
Stay updated with articles, news, and events that are driving technological innovation, transforming industries, and reshaping the digital landscape.
In 1950, Alan Turing, who is considered one of the Fathers of AI, published Computing Machinery and Intelligence...
Today’s business landscape is defined by growing competitiveness, a race toward digitalization, increased volatility, and the challenge of...
We’ve seen it far too often in QA departments: a new testing methodology is announced, automation is on...
The Control-Command and Signalling Technical Specification for Interoperability (CCS TSI) defines the common framework of technical specifications and...
In line with the previous article “Structure, readability and efficiency in code development”, I add some practical tips...
Today’s business landscape is defined by growing competitiveness, a race toward digitalization, increased volatility, and the challenge of...
In line with the previous article “Structure, readability and efficiency in code development”, I add some practical tips...
A common behaviour among data scientists is to learn to develop on Jupyter/Databricks notebooks. However, over time, Notebooks...
In today’s development world, it is common practice to rely heavily on a variety of third-party components, libraries/packages,...
Low Code Dev Business Potential Unleash Your Business Potential with Low-Code Innovation! In today’s ever-evolving business world, speed...
Quality Assurance (QA) stands as a pivotal process, dedicated to elevating the quality of software products, services, or...
We’ve seen it far too often in QA departments: a new testing methodology is announced, automation is on...
Meet The Team – Get to know Irene Durán, Senior QA Engineer and QA Community lead at Capitole...
Optimizing Application Quality with End-to-End Testing: A Deep Dive into Playwright Introduction Playwright, an open-source library by Microsoft,...
What is performance testing? In today’s world, the IT field continues to expand and diversify. Let’s take a...
What mindset drives an effective and efficient software tester? And what distinguishes the agile testing mindset? At Capitole,...
In 1950, Alan Turing, who is considered one of the Fathers of AI, published Computing Machinery and Intelligence...
In the age of digital transformation, few advancements have been as disruptive and rapid as generative artificial intelligence...
The latest advancements of Generative Artificial Intelligence (GenAI) are revolutionizing the world. According to the New York Times,...
To understand a bit about what this article is about, let’s start with the basics: what is AI,...
In the digital era we live in, technological advancement has become a constant that redefines the way businesses...
The Control-Command and Signalling Technical Specification for Interoperability (CCS TSI) defines the common framework of technical specifications and...
Today’s business landscape is defined by growing competitiveness, a race toward digitalization, increased volatility, and the challenge of...
We’ve seen it far too often in QA departments: a new testing methodology is announced, automation is on...
Let’s talk about change management and its importance within tech teams in companies. Digital transformation is the process through...
Power Platform enables individuals and organizations to analyze data, build solutions, and automate processes all within a low-code...
Economic, social, and technological processes are transformed in every industrial revolution. According to experts, we are witnessing the...
In today’s business world, the ability to innovate and adapt quickly is crucial. Design Thinking emerges as a...
Capitole Insights
Access in-depth analysis and expert interviews on the latest tech trends, innovation strategies, and business solutions that will help you lead change in your company and industry.
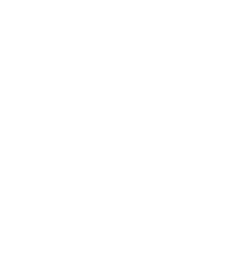
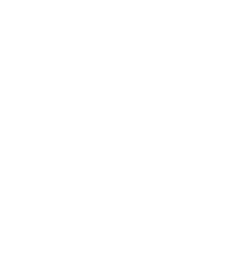
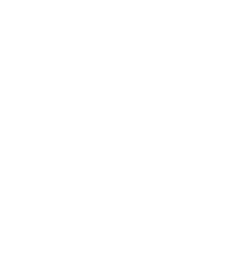
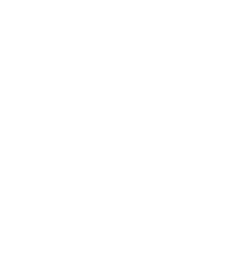
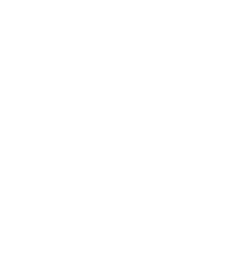
Capitole Tech Talks
Inspiring conversations with industry experts, exploring innovations and strategies that are revolutionizing the future of technology.
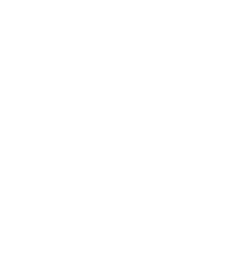
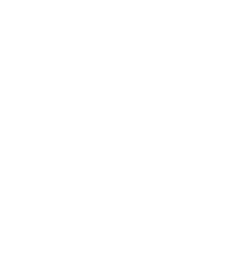
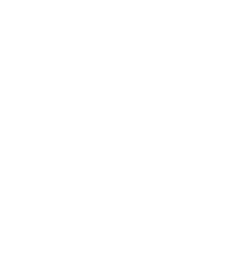
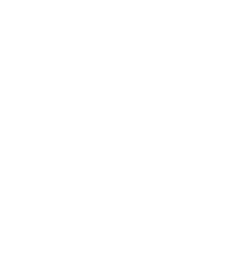
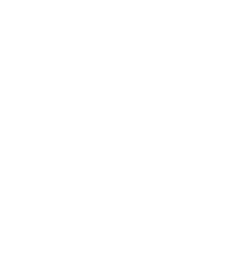